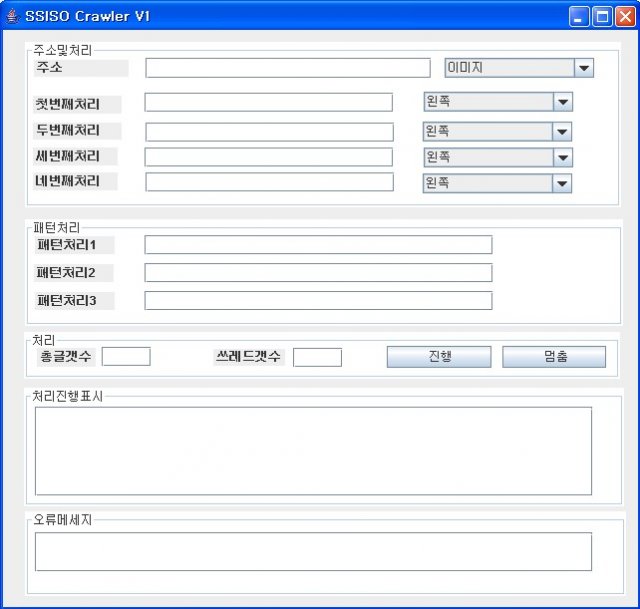 Image size : 640 Ⅹ 609
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.URL;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.JTextField;
import javax.swing.UIManager;
import javax.swing.border.TitledBorder;
class ssisoCrawler_start extends JFrame {
JPanel panel;
JPanel jPanel1;
JPanel jPanel2;
JLabel jLabel4;
JTextField jTextField5;
JLabel jLabel5;
JTextField jTextField6;
JLabel jLabel6;
JTextField jTextField7;
JLabel jLabel7;
JTextField jTextField8;
JPanel jPanel3;
JLabel jLabel8;
JTextField jTextField9;
JLabel jLabel9;
JTextField jTextField10;
JLabel jLabel10;
JTextField jTextField11;
JButton jButton1;
JButton jButton2;
JTextField jTextField12;
JLabel jLabel11;
JLabel jLabel12;
JTextField jTextField13;
JPanel jPanel4;
JPanel jPanel5;
JComboBox jComboBox1;
JComboBox jComboBox2;
JComboBox jComboBox3;
JComboBox jComboBox4;
JLabel jLabel13;
JTextField jTextField14;
JComboBox jComboBox5;
JTextArea jTextArea1;
JScrollPane jTextArea1_scroll;
JTextArea jTextArea2;
JScrollPane jTextArea2_scroll;
private String nextUrl;
public ssisoCrawler_start() {
}
public void processGet(String URL) throws Exception {
int i = 1;
nextUrl = URL;
do {
nextUrl = getArticle_start(nextUrl);
try {
Thread.sleep(500);
} catch (InterruptedException exc) {
}
if (nextUrl.length() < 1) {
i = 0;
}
// System.out.println(nextUrl);
} while (i > 0);
}
public String getArticle_start(String targetURL) {
try {
URL yahoo;
yahoo = new URL(targetURL);
BufferedReader in = new BufferedReader(new InputStreamReader(yahoo
.openStream()));
String inputLine;
StringBuffer pageBuffer = new StringBuffer();
while ((inputLine = in.readLine()) != null)
pageBuffer.append(inputLine);
String pageContent = pageBuffer.toString();
String[] pageContentArray;
Pattern pattern = Pattern
.compile("<img[^>]*[ src=]?[\"']?[^>\"']+[\"']?[^>]*>");
Pattern pattern2 = Pattern.compile("src=[\"|']?([^\"']*)[\"|']");
if (!jTextField6.getText().toString().equals("")) {
if (jComboBox2.getSelectedItem().toString() == "왼쪽") {
System.out.println("왼쪽");
pageContentArray = pageContent.split(jTextField6.getText()
.toString());
pageContent = pageContentArray[0];
} else if (jComboBox2.getSelectedItem().toString() == "오른쪽") {
System.out.println("오른쪽");
pageContentArray = pageContent.split(jTextField6.getText()
.toString());
pageContent = pageContentArray[1];
} else if (jComboBox2.getSelectedItem().toString() == "가운데") {
System.out.println("가운데");
pageContentArray = pageContent.split(jTextField6.getText()
.toString());
pageContent = pageContentArray[1];
}
}
if (!jTextField7.getText().toString().equals("")) {
if (jComboBox3.getSelectedItem().toString() == "왼쪽") {
System.out.println("왼쪽");
pageContentArray = pageContent.split(jTextField7.getText()
.toString());
pageContent = pageContentArray[0];
} else if (jComboBox3.getSelectedItem().toString() == "오른쪽") {
System.out.println("오른쪽");
pageContentArray = pageContent.split(jTextField7.getText()
.toString());
pageContent = pageContentArray[1];
} else if (jComboBox3.getSelectedItem().toString() == "가운데") {
System.out.println("가운데");
pageContentArray = pageContent.split(jTextField7.getText()
.toString());
pageContent = pageContentArray[1];
}
}
Matcher match = pattern.matcher(pageContent);
String sb = null;
int sNum = 1;
jTextArea2.setText("");
while (match.find()) {
sb = match.group(0);
jTextArea2.append(sNum + ": " + sb + "\n");
}
in.close();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private URL verifyUrl(String url) {
// Only allow HTTP URLs.
if (!url.toLowerCase().startsWith("http://"))
return null;
// Verify format of URL.
URL verifiedUrl = null;
try {
verifiedUrl = new URL(url);
} catch (Exception e) {
return null;
}
return verifiedUrl;
}
public void makeComponent() {
UIManager.put("swing.boldMetal", Boolean.FALSE);
setTitle("SSISO Crawler V1");
setBounds(120, 8, 651, 617);
setDefaultCloseOperation(EXIT_ON_CLOSE);
panel = new JPanel();
panel.setLayout(null);
jPanel1 = new JPanel();
jPanel1.setLayout(null);
jPanel1.setFont(new Font("Dialog.plain", 0, 12));
jPanel1.setBackground(new Color(-1));
jPanel1.setBounds(22, 12, 598, 166);
jPanel1.setBorder(new TitledBorder("주소및처리"));
panel.add(jPanel1);
jPanel2 = new JPanel();
jPanel2.setLayout(null);
jPanel2.setFont(new Font("Dialog.plain", 0, 12));
jPanel2.setBackground(new Color(-1));
jPanel2.setBounds(22, 190, 600, 107);
jPanel2.setBorder(new TitledBorder("패턴처리"));
panel.add(jPanel2);
jLabel4 = new JLabel();
jLabel4.setText("주소");
jLabel4.setFont(new Font("Dialog.bold", 1, 12));
jLabel4.setIcon(new ImageIcon(""));
jLabel4.setOpaque(true);
jLabel4.setForeground(new Color(-13421773));
jLabel4.setBackground(new Color(-1118482));
jLabel4.setBounds(9, 18, 95, 17);
jPanel1.add(jLabel4);
jTextField5 = new JTextField();
jTextField5.setText("");
jTextField5.setFont(new Font("Dialog.plain", 0, 12));
jTextField5.setForeground(new Color(-13421773));
jTextField5.setBackground(new Color(-1));
jTextField5.setBounds(121, 16, 287, 21);
jPanel1.add(jTextField5);
jLabel5 = new JLabel();
jLabel5.setText("첫번째처리");
jLabel5.setFont(new Font("Dialog.bold", 1, 12));
jLabel5.setIcon(new ImageIcon(""));
jLabel5.setOpaque(true);
jLabel5.setForeground(new Color(-13421773));
jLabel5.setBackground(new Color(-1118482));
jLabel5.setBounds(8, 54, 89, 18);
jPanel1.add(jLabel5);
jTextField6 = new JTextField();
jTextField6.setText("");
jTextField6.setFont(new Font("Dialog.plain", 0, 12));
jTextField6.setForeground(new Color(-13421773));
jTextField6.setBackground(new Color(-1));
jTextField6.setBounds(120, 51, 250, 20);
jPanel1.add(jTextField6);
jLabel6 = new JLabel();
jLabel6.setText("두번째처리");
jLabel6.setFont(new Font("Dialog.bold", 1, 12));
jLabel6.setIcon(new ImageIcon(""));
jLabel6.setOpaque(true);
jLabel6.setForeground(new Color(-13421773));
jLabel6.setBackground(new Color(-1118482));
jLabel6.setBounds(8, 81, 89, 17);
jPanel1.add(jLabel6);
jTextField7 = new JTextField();
jTextField7.setText("");
jTextField7.setFont(new Font("Dialog.plain", 0, 12));
jTextField7.setForeground(new Color(-13421773));
jTextField7.setBackground(new Color(-1));
jTextField7.setBounds(121, 81, 250, 20);
jPanel1.add(jTextField7);
jLabel7 = new JLabel();
jLabel7.setText("세번째처리");
jLabel7.setFont(new Font("Dialog.bold", 1, 12));
jLabel7.setIcon(new ImageIcon(""));
jLabel7.setOpaque(true);
jLabel7.setForeground(new Color(-13421773));
jLabel7.setBackground(new Color(-1118482));
jLabel7.setBounds(8, 106, 85, 18);
jPanel1.add(jLabel7);
jTextField8 = new JTextField();
jTextField8.setText("");
jTextField8.setFont(new Font("Dialog.plain", 0, 12));
jTextField8.setForeground(new Color(-13421773));
jTextField8.setBackground(new Color(-1));
jTextField8.setBounds(120, 106, 250, 20);
jPanel1.add(jTextField8);
jPanel3 = new JPanel();
jPanel3.setLayout(null);
jPanel3.setFont(new Font("Dialog.plain", 0, 12));
jPanel3.setBackground(new Color(-1));
jPanel3.setBounds(21, 303, 598, 47);
jPanel3.setBorder(new TitledBorder("처리"));
panel.add(jPanel3);
jLabel8 = new JLabel();
jLabel8.setText("패턴처리1");
jLabel8.setFont(new Font("Dialog.bold", 1, 12));
jLabel8.setIcon(new ImageIcon(""));
jLabel8.setOpaque(true);
jLabel8.setForeground(new Color(-13421773));
jLabel8.setBackground(new Color(-1118482));
jLabel8.setBounds(10, 17, 80, 18);
jPanel2.add(jLabel8);
jTextField9 = new JTextField();
jTextField9.setText("");
jTextField9.setFont(new Font("Dialog.plain", 0, 12));
jTextField9.setForeground(new Color(-13421773));
jTextField9.setBackground(new Color(-1));
jTextField9.setBounds(120, 16, 350, 20);
jPanel2.add(jTextField9);
jLabel9 = new JLabel();
jLabel9.setText("패턴처리2");
jLabel9.setFont(new Font("Dialog.bold", 1, 12));
jLabel9.setIcon(new ImageIcon(""));
jLabel9.setOpaque(true);
jLabel9.setForeground(new Color(-13421773));
jLabel9.setBackground(new Color(-1118482));
jLabel9.setBounds(9, 45, 80, 18);
jPanel2.add(jLabel9);
jTextField10 = new JTextField();
jTextField10.setText("");
jTextField10.setFont(new Font("Dialog.plain", 0, 12));
jTextField10.setForeground(new Color(-13421773));
jTextField10.setBackground(new Color(-1));
jTextField10.setBounds(120, 44, 350, 20);
jPanel2.add(jTextField10);
jLabel10 = new JLabel();
jLabel10.setText("패턴처리3");
jLabel10.setFont(new Font("Dialog.bold", 1, 12));
jLabel10.setIcon(new ImageIcon(""));
jLabel10.setOpaque(true);
jLabel10.setForeground(new Color(-13421773));
jLabel10.setBackground(new Color(-1118482));
jLabel10.setBounds(10, 73, 80, 18);
jPanel2.add(jLabel10);
jTextField11 = new JTextField();
jTextField11.setText("");
jTextField11.setFont(new Font("Dialog.plain", 0, 12));
jTextField11.setForeground(new Color(-13421773));
jTextField11.setBackground(new Color(-1));
jTextField11.setBounds(120, 72, 350, 20);
jPanel2.add(jTextField11);
jButton1 = new JButton();
jButton1.setText("진행");
jButton1.setFont(new Font("Dialog.plain", 0, 12));
jButton1.setIcon(new ImageIcon(""));
jButton1.setForeground(new Color(-13421773));
jButton1.setBounds(364, 14, 105, 22);
jPanel3.add(jButton1);
jButton1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String link = jTextField5.getText().toString();
URL verifiedLink = verifyUrl(link);
if (verifiedLink != null) {
try {
processGet(link);
} catch (Exception e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
} else {
System.out.println("잘못된 주소입니다");
}
}
});
jButton2 = new JButton();
jButton2.setText("멈춤");
jButton2.setFont(new Font("Dialog.plain", 0, 12));
jButton2.setIcon(new ImageIcon(""));
jButton2.setForeground(new Color(-13421773));
jButton2.setBounds(480, 14, 104, 22);
jPanel3.add(jButton2);
jTextField12 = new JTextField();
jTextField12.setText("");
jTextField12.setFont(new Font("Dialog.plain", 0, 12));
jTextField12.setForeground(new Color(-13421773));
jTextField12.setBackground(new Color(-1));
jTextField12.setBounds(78, 15, 50, 20);
jPanel3.add(jTextField12);
jLabel11 = new JLabel();
jLabel11.setText("총글갯수");
jLabel11.setFont(new Font("Dialog.bold", 1, 12));
jLabel11.setIcon(new ImageIcon(""));
jLabel11.setOpaque(true);
jLabel11.setForeground(new Color(-13421773));
jLabel11.setBackground(new Color(-1118482));
jLabel11.setBounds(13, 16, 59, 19);
jPanel3.add(jLabel11);
jLabel12 = new JLabel();
jLabel12.setText("쓰레드갯수");
jLabel12.setFont(new Font("Dialog.bold", 1, 12));
jLabel12.setIcon(new ImageIcon(""));
jLabel12.setOpaque(true);
jLabel12.setForeground(new Color(-13421773));
jLabel12.setBackground(new Color(-1118482));
jLabel12.setBounds(190, 17, 72, 17);
jPanel3.add(jLabel12);
jTextField13 = new JTextField();
jTextField13.setText("");
jTextField13.setFont(new Font("Dialog.plain", 0, 12));
jTextField13.setForeground(new Color(-13421773));
jTextField13.setBackground(new Color(-1));
jTextField13.setBounds(270, 16, 50, 20);
jPanel3.add(jTextField13);
jPanel4 = new JPanel();
jPanel4.setLayout(null);
jPanel4.setFont(new Font("Dialog.plain", 0, 12));
jPanel4.setBackground(new Color(-1));
jPanel4.setBounds(22, 483, 603, 85);
jPanel4.setBorder(new TitledBorder("오류메세지"));
panel.add(jPanel4);
jPanel5 = new JPanel();
jPanel5.setLayout(null);
jPanel5.setFont(new Font("Dialog.plain", 0, 12));
jPanel5.setBackground(new Color(-1));
jPanel5.setBounds(21, 359, 602, 119);
jPanel5.setBorder(new TitledBorder("처리진행표시"));
panel.add(jPanel5);
jComboBox1 = new JComboBox();
jComboBox1.addItem("이미지");
jComboBox1.addItem("플래쉬");
jComboBox1.addItem("동영상");
jComboBox1.setFont(new Font("Dialog.plain", 0, 12));
jComboBox1.setForeground(new Color(-13421773));
jComboBox1.setBackground(new Color(-1118482));
jComboBox1.setBounds(421, 16, 150, 20);
jPanel1.add(jComboBox1);
jComboBox2 = new JComboBox();
jComboBox2.addItem("왼쪽");
jComboBox2.addItem("오른쪽");
jComboBox2.addItem("가운데");
jComboBox2.setFont(new Font("Dialog.plain", 0, 12));
jComboBox2.setForeground(new Color(-13421773));
jComboBox2.setBackground(new Color(-1118482));
jComboBox2.setBounds(400, 50, 150, 20);
jPanel1.add(jComboBox2);
jComboBox3 = new JComboBox();
jComboBox3.addItem("왼쪽");
jComboBox3.addItem("오른쪽");
jComboBox3.addItem("가운데");
jComboBox3.setFont(new Font("Dialog.plain", 0, 12));
jComboBox3.setForeground(new Color(-13421773));
jComboBox3.setBackground(new Color(-1118482));
jComboBox3.setBounds(399, 80, 150, 20);
jPanel1.add(jComboBox3);
jComboBox4 = new JComboBox();
jComboBox4.addItem("왼쪽");
jComboBox4.addItem("오른쪽");
jComboBox4.addItem("가운데");
jComboBox4.setFont(new Font("Dialog.plain", 0, 12));
jComboBox4.setForeground(new Color(-13421773));
jComboBox4.setBackground(new Color(-1118482));
jComboBox4.setBounds(400, 106, 150, 20);
jPanel1.add(jComboBox4);
jLabel13 = new JLabel();
jLabel13.setText("네번째처리");
jLabel13.setFont(new Font("Dialog.bold", 1, 12));
jLabel13.setIcon(new ImageIcon(""));
jLabel13.setOpaque(true);
jLabel13.setForeground(new Color(-13421773));
jLabel13.setBackground(new Color(-1118482));
jLabel13.setBounds(8, 131, 85, 18);
jPanel1.add(jLabel13);
jTextField14 = new JTextField();
jTextField14.setText("");
jTextField14.setFont(new Font("Dialog.plain", 0, 12));
jTextField14.setForeground(new Color(-13421773));
jTextField14.setBackground(new Color(-1));
jTextField14.setBounds(121, 131, 250, 20);
jPanel1.add(jTextField14);
jComboBox5 = new JComboBox();
jComboBox5.addItem("왼쪽");
jComboBox5.addItem("오른쪽");
jComboBox5.addItem("가운데");
jComboBox5.setFont(new Font("Dialog.plain", 0, 12));
jComboBox5.setForeground(new Color(-13421773));
jComboBox5.setBackground(new Color(-1118482));
jComboBox5.setBounds(399, 132, 150, 20);
jPanel1.add(jComboBox5);
jTextArea1 = new JTextArea();
jTextArea1_scroll = new JScrollPane(jTextArea1);
jTextArea1.setText("");
jTextArea1.setFont(new Font("Dialog.plain", 0, 12));
jTextArea1.setForeground(new Color(-13421773));
jTextArea1.setBackground(new Color(-1));
jTextArea1_scroll.setBounds(10, 21, 560, 40);
jPanel4.add(jTextArea1_scroll);
jTextArea2 = new JTextArea();
jTextArea2_scroll = new JScrollPane(jTextArea2);
jTextArea2.setText("");
jTextArea2.setFont(new Font("Dialog.plain", 0, 12));
jTextArea2.setForeground(new Color(-13421773));
jTextArea2.setBackground(new Color(-1));
jTextArea2_scroll.setBounds(11, 19, 560, 90);
jPanel5.add(jTextArea2_scroll);
getContentPane().add(panel, BorderLayout.CENTER);
setVisible(true);
}
}
public class ssisoCrawler {
public static void main(String[] args) {
ssisoCrawler_start GUI_Interface = new ssisoCrawler_start();
GUI_Interface.makeComponent();
}
}
현재 진행중입니다.
썸네일 만드는 부분은 이전에 올린 소스 참고하시면 됩니다
썸네일 만드는 부분에서 JAI API가 다양한 포맷이 지원이 안되 자꾸 오류가 발생하여
새로운 방법을 찾고 있습니다.
|